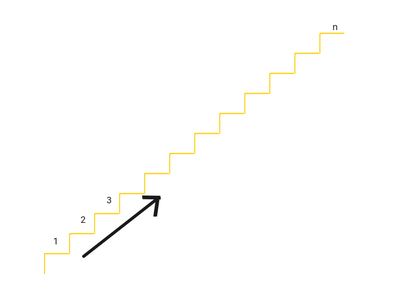
The Story begins with a Problem
Yesterday, I was solving a very famous DP problem, the climbing stairs.
The problem is quite simple. You are climbing a staircase. It takes n steps to reach to the top. Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?
Solution
The solution is also pretty straight forward.
Suppose we have climbed up some steps and we are left with some i steps to reach the top. Let’s say, NoOfWays(i) be a function that returns the number of ways to climb i steps to reach the top.

If we climb by 1 step, then we are left with (i-1) steps. Using the above formula, the number of ways to climb (i -1) steps = NoOfWays(i-1).
Similarly, if we climb by 2 steps, then we are left with (i-2) steps, and using the formula, the number of ways to climb (i -2) steps = NoOfWays(i-2).
Since we can climb in one of the two fashions only so the number of ways to climb i steps is given as follows

For the base cases, we know
NoOfWays(1) = 1 and NoOfWays(2) = 2, therefore we can say

With this recurrence relation, we can determine the number of ways to climb n steps to reach the top. Formally this solves the problem.
Now the solution and everything is fine but what is so special about this problem?
The answer is the result.

On reversing the above equation, we can state that

Using the above representation of the Fibonacci numbers we can prove various identities and rules associated with the Fibonacci sequence.
Let’s say, Fib(n) represents the nᵗʰ Fibonacci Number.
The Convolution Theorem

Clearly the left-hand side of the equation represents the number of ways to climb the (n + k) stairs.
The task of climbing (n + k) stairs can also be completed in one of the two ways.
- Case #1: If we step onto the nth step every time we climb
- Case #2: If we skip the nth step every time
In Case #1,
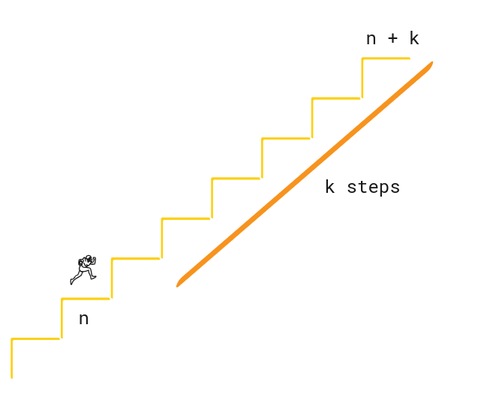
Since we stepped onto the nᵗʰ step. Then we must have climbed n steps in NoOfWays(n) ways, and are left with k steps to climb further.
Number of ways to climb = NoOfWays(n) * NoOfWays(k)
In Case #2,

Since we skipped the nᵗʰ step. Then we must have climbed to the (n-1)ᵗʰ step and jumped to the (n + 1)ᵗʰ step.
Hence, Number of ways to climb = NoOfWays(n-1) * NoOfWays(k-1)
The cases discussed above are mutually exclusive (i.e. there can’t be a path which exists in both of the cases simultaneously) and exhaustive to the space of possibility (i.e. the total number of ways to climb the whole staircase)
We can say that,

Since NoOfWays(n) = Fib(n), therefore

This proves the Convolution Theorem of Fibonacci Number.
Further insight
Putting k = n and k = n +1 in the above equation,

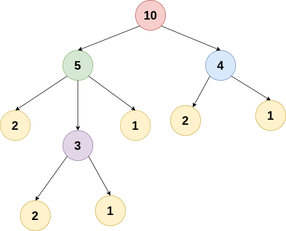
T(n) = 2*T(n/2) + O(1) when n is even
T(n) = 3*T(n/2) + O(1) when n is odd
Using the above results we can compute nth Fibonacci Number in O(log²N).
Similarly, we can derive the following summations on the Fibonacci Sequence using this representation method (I assume this, I haven’t thought of it yet)
- Running Sum, Fib(n) + Fib(n-1) + Fib(n-2) … + Fib(0) = Fib(n+2) -1
(UPDATE: link to my intrepetation of this formula is available here) - Sum of Even terms, Fib(0) + Fib(2) + Fib(4) …. + Fib(2n) = Fib(2n+1)
Some other representations that I found useful
- Fib(n) = Number of ways to get a sum (= n) using only 1s and 2s.
- Fib(n) = Number of ways to tile an n x 1 board using a 1 x 1 square and 2 x 1 domino (another DP problem)

Towards the End…
All of this hit me while trying a question, that was easy and I already knew the logic and solution to but, till then I haven’t seen it the other way round. Then, I looked up for some popular properties on Google and tried it on them, and it worked out.
The idea isn’t unique and there might be some better representation of the sequence, that must have already been discussed before. But I found it cool, so I shared it with you. I also believe that representation like this helps in solving similar problems with complex modifications, that are difficult to decode using only mathematical equations. I hope you like the idea.
That’s my part
Signing off
Comments
Post a Comment